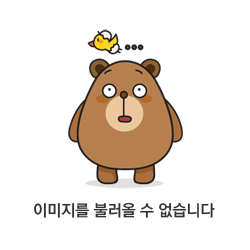
✔️ React Native Components and apis
https://reactnative.dev/docs/components-and-apis
Core Components and APIs · React Native
React Native provides a number of built-in Core Components ready for you to use in your app. You can find them all in the left sidebar (or menu above, if you are on a narrow screen). If you're not sure where to get started, take a look at the following cat
reactnative.dev
✔️ Expo SDK
https://docs.expo.dev/versions/latest/?redirected
Reference
Expo is an open-source platform for making universal native apps for Android, iOS, and the web with JavaScript and React.
docs.expo.dev
✔️ Flex
export default function App() {
return (
<View style={{flex:1}}>
<View style={{flex:1, backgroundColor:"tomato"}}></View>
<View style={{flex:3, backgroundColor:"teal"}}></View>
<View style={{flex:1, backgroundColor:"orange"}}></View>
</View>
);
}
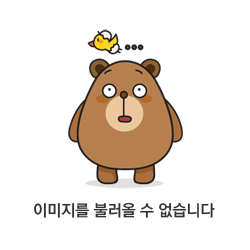
웹과는 다르게 React Native 에서는 flex 를 이용해서 비율로 레이아웃을 설정할 수 있다.
✔️ Component props
import { StatusBar } from 'expo-status-bar';
import {ScrollView, StyleSheet, Text, View, Dimensions} from 'react-native';
const { width: SCREEN_WIDTH } = Dimensions.get("window");
export default function App() {
return (
<View style={styles.container}>
<View style={styles.city}>
<Text style={styles.cityName}>Seoul</Text>
</View>
<ScrollView
pagingEnabled
horizontal
showsHorizontalScrollIndicator={false}
contentContainerStyle={styles.weather}>
<View style={styles.day}>
<Text style={styles.temperature}>27</Text>
<Text style={styles.description}>Sunny</Text>
</View>
<View style={styles.day}>
<Text style={styles.temperature}>27</Text>
<Text style={styles.description}>Sunny</Text>
</View>
<View style={styles.day}>
<Text style={styles.temperature}>27</Text>
<Text style={styles.description}>Sunny</Text>
</View>
<View style={styles.day}>
<Text style={styles.temperature}>27</Text>
<Text style={styles.description}>Sunny</Text>
</View>
</ScrollView>
<StatusBar style="light"></StatusBar>
</View>
);
}
여기서 확인해봐야할 부분은 React Native 에서 제공하는 Component 와 그 안에 props 를 활용해서 다양한 기능을 넣었다는 점이다.
우선 Dimensions Component 를 활용해 자신의 모바일 기기 width 값을 가져와서 style 지정에 활용했고,
ScrollView Component 의 다양한 props 를 활용해서 가로배치, 페이지네이션 등 기능을 구현했다는 점이다.
'컴퓨터 프로그래밍 > React Native' 카테고리의 다른 글
[React Native] Todo 어플리케이션 (0) | 2025.03.30 |
---|---|
[React Native] 날씨 어플리케이션 (0) | 2025.03.29 |
[React Native] 개념 및 작동원리, 환경세팅 (0) | 2025.03.26 |